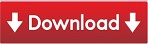
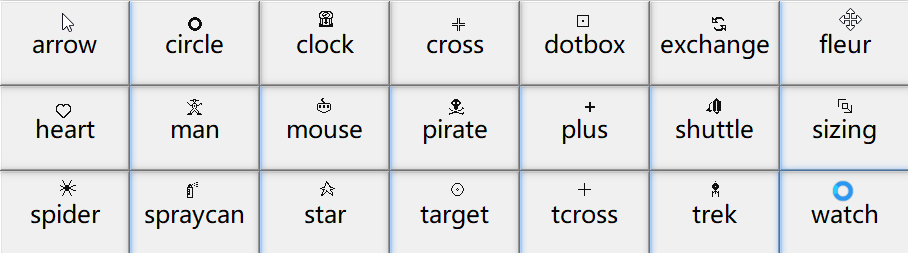
To set the selected item, you can pass the index of the desired item to the current function.
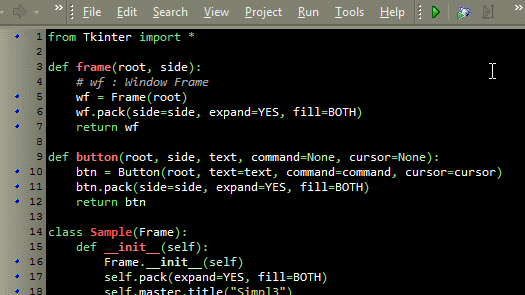
from tkinter import *Īs you can see, we add the combobox items using the tuple. Then you can add your values to the combobox. To add a combobox widget, you can use the Combobox class from ttk library like this: from tkinter.ttk import * Now, you won’t be able to enter any text. To disable the entry widget, you can set the state property to disabled: txt = Entry(window,width=10, state='disabled') That’s super easy, all we need to do is to call focus function like this: txt.focus()Īnd when you run your code, you will notice that the entry widget has the focus so you can write your text right away. If you click the button and there is a text on the entry widget, it will show “Welcome to” concatenated with the entered text.Īnd this is the complete code: from tkinter import *Įvery time we run the code, we need to click on the entry widget to set focus to write the text, what about setting the focus automatically? Set focus to the entry widget
#Python tkinter icursor example code
So we can write this code to our clicked function like this: def clicked(): Now, if you click the button, it will show the same old message, what about showing the entered text on the Entry widget?įirst, you can get entry text using get function. Lbl.configure(text="Button was clicked !!")ītn = Button(window, text="Click Me", command=clicked) So our window will be like this: from tkinter import * Let’s start by adding the button to the window, the button is created and added to the window the same as the label: btn = Button(window, text="Click Me")
#Python tkinter icursor example how to
Let’s try adding more GUI widgets like buttons and see how to handle the button click event. The above line sets the window width to 350 pixels and the height to 200 pixels.

We can set the default window size using geometry function like this: window.geometry('350x200') Great, but the window is so small, we can even see the title, what about setting the window size? Setting window size Note that the font parameter can be passed to any widget to change its font not labels only. To do so, you can pass the font parameter like this: lbl = Label(window, text="Hello", font=("Arial Bold", 50)) You can set the label font so you can make it bigger and maybe bold. Without calling the grid function for the label, it won’t show up. So the complete code will be like this: from tkinter import * Then we will set its position on the form using the grid function and give it the location like this: lbl.grid(column=0, row=0) To add a label to our previous example, we will create a label using the label class like this: lbl = Label(window, text="Hello")
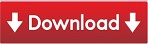